In my last post, I went over the design of a Colpitts crystal oscillator design that put out a moderately clean 7 MHz signal. In order to match the output impedance to 50 Ω, an NPN feedback pair (at least that’s what I’m calling it) was designed. While meeting the specs for driving an ADE-1 mixer, it consumed an unnecessary amount of current. I’ve been designing a tremolo effect recently (which I should be making on post on as well in the future) where I used a BS170 MOSFET to amplitude modulate an incoming audio signal. Without going into too much detail, I decided to adjust the DC bias point of an emitter-follower to sit at the threshold voltage of the BS170 to prevent from having to have a DC block immediately followed by another DC bias point. While looking at different transmitter designs on Homebrew RF Circuit Design Ideas, I came across a class C amplifier that used a similar technique to what I was doing in the tremolo effect combined with a Pierce oscillator. I did some experimentation and came up with the following circuit.
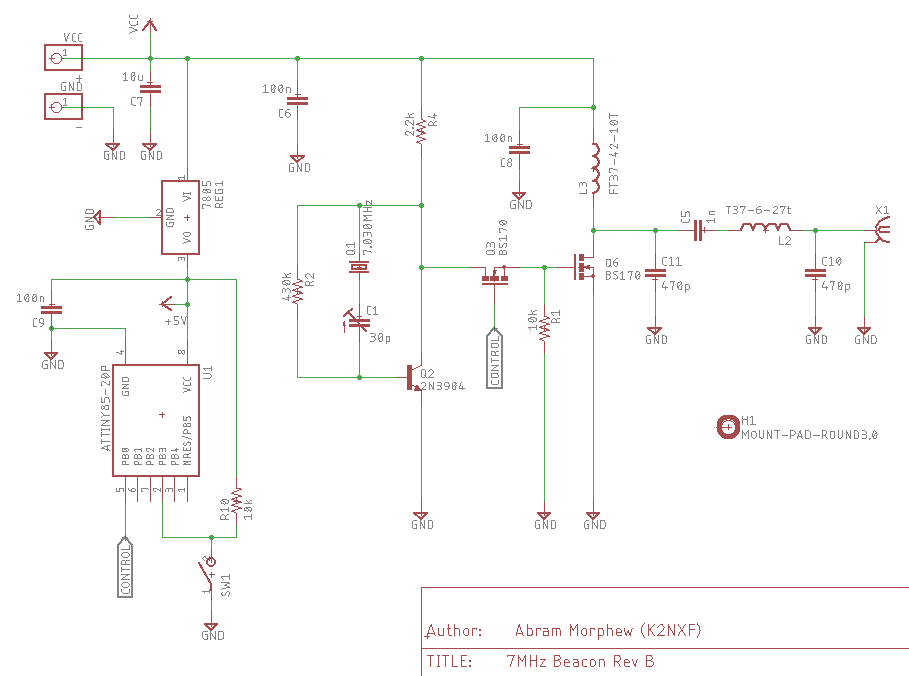
To be fair, this schematic is revision B, and it hasn’t been built yet. Revision A, however, is pretty much the same thing except R1 is omitted and the drain of Q3 connects to the source of Q6. The ATTiny85 takes the single-throw switch as the only input. When you turn it on, the switch acts like a standard on-off keyer for banging out morse code. If you hold the key down for 5 seconds, it changes to a beacon mode and starts tapping out my call sign. Holding the key down again changes operation into pulse mode with a frequency around 1 kHz. In pulse mode, you can actually pick up the signal on a standard AM receiver as seen in the demo video. The transmitter itself puts out 28 dBm running on a 12V supply and is around 82% efficient.
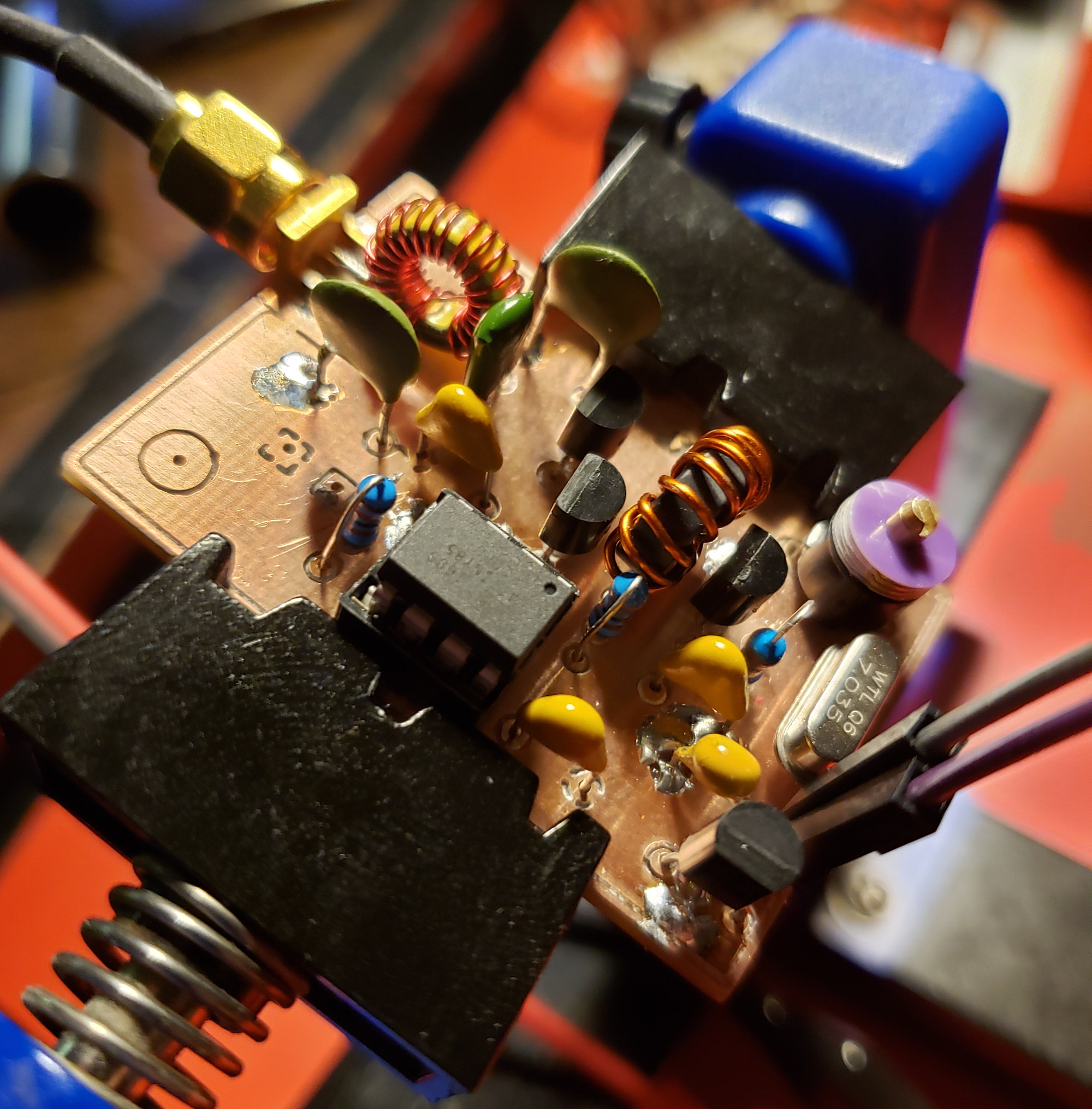
The PCB layout came out pretty quickly. It was the first time I have ever done double-sided PCB etching. Overall, I think it came out pretty well. There was small offset as you can see from the placement of the drill holes, but no harm, no foul. Performance was even a little better than the prototype. I mounted the board inside a Hammond 1590A enclosure and made a short demonstration video. It’s extremely simple, but I think it will be a useful piece of a larger project that I’m working on. It can also be easily adapted to a number of frequencies using a different crystal or loading Q2 to act as a frequency multiplier. I did experiment with this somewhat and was able to produce fairly strong second and third harmonics at the output. That’s about as far as I got though since I got distracted making theremin type sounds on my shortwave radio receiver.
#define cbi(sfr, bit) (_SFR_BYTE(sfr) &= ~_BV(bit)) #define sbi(sfr, bit) (_SFR_BYTE(sfr) |= _BV(bit)) #define CTL 0 // TX enable pin #define SW 3 // input switch pin #define N 10 // N periods in monopulse mode bool sw = LOW; bool trig = LOW; // monopulse trigger status bool tx = LOW; // flag for TX enable bool keyer = LOW; // flag for start of key press int mode = 0; // modes: 0 => Key mode (default), 1 => ID mode, 2 => Monopulse int dit = 50; // delay time for dit in ms int dash = 150; // delay time for dash in ms unsigned long hold = 5e6; // max hold value in microseconds unsigned long pause = 1e7; // max pause between IDs in microseconds unsigned long start = 0; // start time in microseconds unsigned long last = 0; // time of last ID; long c = 0; // count variable for mode change void setup() { pinMode(CTL,OUTPUT); pinMode(SW, INPUT); cli(); /*--- TIMER1 CONFIG ---*/ TCCR1 = 0b01101000; GTCCR = 0b00100000; TCCR0A = 0b00100000; TCCR0B = 0b00001011; // last 3 bits set prescalar for Timer0 cbi(TIFR,OCF1A); sbi(TIMSK,OCIE1A); OCR1A = 128; sei(); /* --- interrupt enable GIMSK = 0b00100000; // turns on pin change interrupts PCMSK = 0b00001000; // turn on interrupts on pins PB3 sei(); */ } void loop() { /* if (sw == LOW) { start = micros(); if (keyer == HIGH) { modeChk(); } else { keyer = HIGH; } } else { keyer = LOW; } */ // perform function based on mode switch(mode) { case 0: if (sw == LOW) { digitalWrite(CTL, HIGH); } else { digitalWrite(CTL, LOW); } break; case 1: if (last == 0) { id(); } else if ((micros() - last) > pause) { id(); } break; case 2: if (sw == LOW) { pulse(); } break; } trig = LOW; // monopulse trigger reset } ISR(TIMER1_COMPA_vect) { sw = digitalRead(SW); if (sw == LOW) { if (keyer == HIGH) { modeChk(); } else { start = micros(); keyer = HIGH; trig = HIGH; } } else { keyer = LOW; } } void modeChk() { if ((micros() - start) > hold) { if (mode < 2) { mode++; } else { mode = 0; } start = micros(); stat(); delay(1000); } } void pulse() { // pulse TX on and off N times for(int n = 0; n < N; n++) { digitalWrite(CTL,HIGH); delayMicroseconds(500); digitalWrite(CTL,LOW); delayMicroseconds(500); } } void stat() { // tap out mode number in morse code for(int n = 0; n < mode; n++) { digitalWrite(CTL,HIGH); delay(dit); digitalWrite(CTL,LOW); delay(dit); } for(int n = 0; n < (5 - mode); n++) { digitalWrite(CTL,HIGH); delay(dash); digitalWrite(CTL,LOW); delay(dit); } } void id() { // tap out ID for K2NXF // K digitalWrite(CTL,HIGH); delay(dash); digitalWrite(CTL,LOW); delay(dit); digitalWrite(CTL,HIGH); delay(dit); digitalWrite(CTL,LOW); delay(dit); digitalWrite(CTL,HIGH); delay(dash); digitalWrite(CTL,LOW); delay(dash); // 2 digitalWrite(CTL,HIGH); delay(dit); digitalWrite(CTL,LOW); delay(dit); digitalWrite(CTL,HIGH); delay(dit); digitalWrite(CTL,LOW); delay(dit); digitalWrite(CTL,HIGH); delay(dash); digitalWrite(CTL,LOW); delay(dit); digitalWrite(CTL,HIGH); delay(dash); digitalWrite(CTL,LOW); delay(dit); digitalWrite(CTL,HIGH); delay(dash); digitalWrite(CTL,LOW); delay(dash); // N digitalWrite(CTL,HIGH); delay(dash); digitalWrite(CTL,LOW); delay(dit); digitalWrite(CTL,HIGH); delay(dit); digitalWrite(CTL,LOW); delay(dash); // X digitalWrite(CTL,HIGH); delay(dash); digitalWrite(CTL,LOW); delay(dit); digitalWrite(CTL,HIGH); delay(dit); digitalWrite(CTL,LOW); delay(dit); digitalWrite(CTL,HIGH); delay(dit); digitalWrite(CTL,LOW); delay(dit); digitalWrite(CTL,HIGH); delay(dash); digitalWrite(CTL,LOW); delay(dash); // F digitalWrite(CTL,HIGH); delay(dit); digitalWrite(CTL,LOW); delay(dit); digitalWrite(CTL,HIGH); delay(dit); digitalWrite(CTL,LOW); delay(dit); digitalWrite(CTL,HIGH); delay(dash); digitalWrite(CTL,LOW); delay(dit); digitalWrite(CTL,HIGH); delay(dit); digitalWrite(CTL,LOW); delay(dash); // new word digitalWrite(CTL,LOW); delay(dash); last = micros(); // store current time at end of ID }