Someone contacted me recently about using an ATMega328 to generate a 200 kHz clock signal for a BBD analog delay chip. I finally had a few minutes today to sit down and ensure that this code works. Varying the OCR0A value acts as a frequency adjustment on the output following the formula: f = 16e6 / (4 * OCR0A) where OCR0A != 0. With a value of 0, the output frequency is roughly 8 MHz. It seemed fairly stable enough at this frequency, but I imagine that it would start having issues with more instructions. Either way, it makes for a very usable clock signal in the kHz range. I’ll try it out on a BBD hopefully one day myself and see.
C++
x
// ===== 200 kHz Clock Signal Generator ==== //
int pin = 6;
byte data = LOW;
void setup() {
setupTimer();
pinMode(pin, OUTPUT);
// f = 16e6 / (4 * OCR0A)
OCR0A = 20; // varies CLK frequency: 0 => 8 MHz, 255 => 16 kHz
digitalWrite(pin,data);
}
void loop() {
}
void setupTimer() {
cli();
/*--- TIMER0 CONFIG ---*/
TCCR0A = 0b11000001;
TCCR0B = 0b00001001; // last 3 bits set prescalar for Timer0
TIMSK0 = 0b00000010; // set OCIE0A high
TIFR0 = 0b00000010; // set OCF0A high
sei();
}
ISR(TIMER0_COMPA_vect) {
data = !data;
digitalWrite(pin, data);
}
</pre>
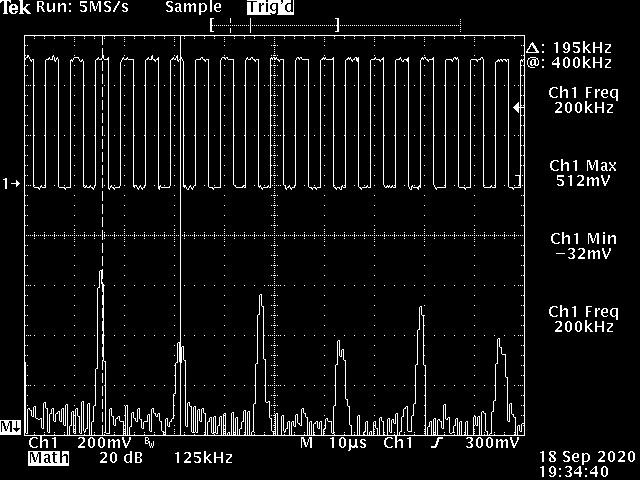
Leave a Reply
You must be logged in to post a comment.